1> Change the DataTemplate to HierarchicalDataTemplate for the second level
2> Add a new DataTemplate to show the third level
The classes used in the sample:
Loading data in Window class:public class Category{public string Name{ get; set; }public List<Company> ItemList{ get; set; }}public class Company{public string Name{ get; set; }public List<Product> Products{ get; set; }}public class Product{public string Name{ get; set; }}
Modifying the XAML:private void Load(){Company cMS = new Company { Name = "Microsoft", Products = new List<Product>() { new Product { Name = "Windows" }, new Product { Name = "MS Office" } } };Company cApple = new Company { Name = "Apple", Products = new List<Product>() { new Product { Name = "iPhone" }, new Product { Name = "iPad" }, new Product { Name = "Mac" } } };Company cIntel = new Company { Name = "Intel", Products = new List<Product>() { new Product { Name = "i5 Processor" }, new Product { Name = "i3 Processor" } } };Company cAMD = new Company { Name = "AMD", Products = new List<Product>() { new Product { Name = "Processor" } } };Category cSoftware = new Category { Name = "Software Companies", ItemList = new List<Company>() { cMS, cApple } };Category cHardware = new Category { Name = "Hardware Companies", ItemList = new List<Company>() { cIntel, cAMD } };List<Category> categories = new List<Category>();categories.Add(cSoftware);categories.Add(cHardware);tv.DataContext = categories;}
Result:<TreeView Name="tv" ItemsSource="{Binding}"><TreeView.Resources><DataTemplate x:Key="productsTemplate"><TextBlock Text="{Binding Path=Name}" /></DataTemplate><HierarchicalDataTemplate x:Key="companyTemplate" ItemsSource="{Binding Path=Products}" ItemTemplate="{StaticResource ResourceKey=productsTemplate}"><TextBlock Text="{Binding Path=Name}" /></HierarchicalDataTemplate></TreeView.Resources><TreeView.ItemTemplate><HierarchicalDataTemplate ItemsSource="{Binding Path=ItemList}" ItemTemplate="{StaticResource ResourceKey=companyTemplate}"><Border BorderBrush="BurlyWood" BorderThickness="2"><TextBlock Text="{Binding Path=Name}" /></Border></HierarchicalDataTemplate></TreeView.ItemTemplate></TreeView>
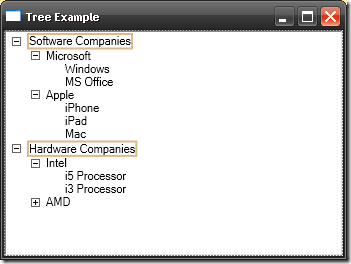
Great example, thanks
ReplyDeleteThanks!
ReplyDelete